Guest post from Scott Page
Here are screenshots of an app that I made using NSBasic to send out SMS Text alerts to our staff. Most of our employees do not like to carry both pagers and cell phones and prefer to receive messages via SMS on their phones. Our CAD (Computer Aided Dispatch) system does not provide for this functionality. My first attempt was to create email groups with the cell phone number and the carrier (5415551212@vtext.com). This worked but had its own set of problems. Some people would get messages before others and there could be a great delay in between the message being sent and when it was received. I believe this has a great deal to do with how the cell providers handle email submissions. Using this method I had to know each person’s cell provider and if they changed they had to tell me so I could make the changes.
I decided to use SMSified as a SMS provider and used their REST Api to interface with my app. This greatly speeds up the rate at which the text messages are received and provides a consistent phone number that is tied to the alerts we send out (before the messages would be from whoever sent the message).
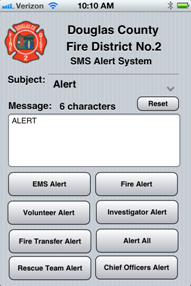
This is the main screen. The user (our Chief Officers) can choose a subject for the alert and then type the message they want to send. The character counter displays how many characters they have used and when the limit is reached a message box alerts them. After typing the message the user presses the button for the group they want to send the message to.
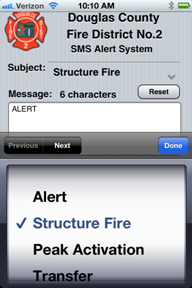
We have both paid and volunteer staff in our department. On a Fire Alert a message box pops up to ask if the volunteer group should be included in the alert. I added the Confirm Send as a way to cancel out of the alert since the SMS message is sent as soon as the group’s button is pressed. When you confirm sending or cancel a message will confirm that the message was sent or aborted. The last screenshot is how it looks on the phone when it is received.
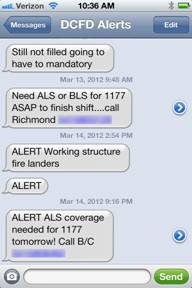
To send the message to SMSified is just a couple of php files on my web server which I got from their tutorials. This is the sendsms.php file:
<?php // Include the SMSifed class. require 'smsified.class.php'; // Text info from application $address = $_POST['address']; $message = $_POST['message']; // SMSified Account settings. $username = "Username"; $password = "Password"; $senderAddress = "5415551212"; try { // Create a new instance of the SMSified object. $sms = new SMSified($username, $password); // Send an SMS message and decode the JSON response from SMSified. $response = $sms->sendMessage($senderAddress, $address, $message); $responseJson = json_decode($response); var_dump($response); } catch (SMSifiedException $ex) { echo $ex->getMessage(); } // Now, redirect back to the client, adding some information for it. header("HTTP/1.1 303 See Other"); header("Location: http://your_url");
I took out my information in the php code. When a user presses one of the buttons it posts to the url on the webserver. Currently it is used for alert notifications only so I don’t handle sms responses but when I get more time I plan to make it so that the receiver of the message can reply back that they are responding.
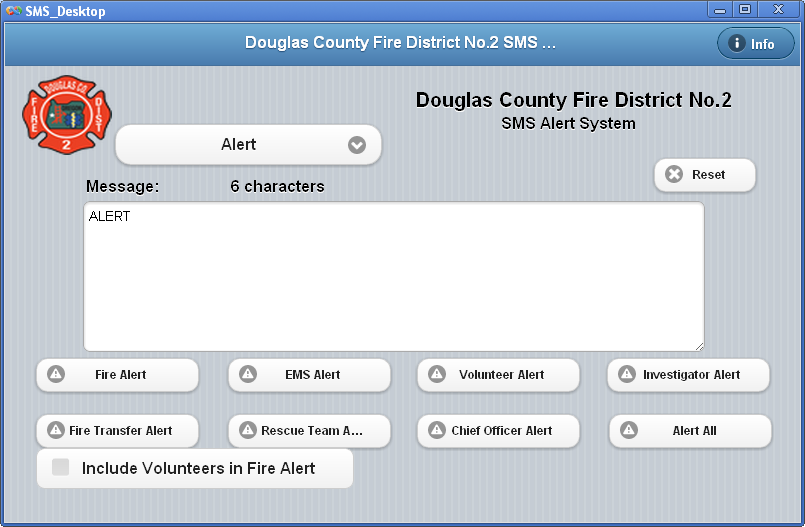
The other cool thing about NSBasic is that I was able to use the same code and make a desktop version that can be used by Dispatch. I made the desktop version a chrome extension and installed it on their computers.