You may have read the recent blog entry called Ajax made Simple. Today we’re going to extend that a bit and talk about using the POST method.
The last blog post discussed the simplest Ajax call, which sends data to the server by adding it to the end of the URL, a method called, rather misleadingly, GET. But what if we want to send more data? GET has a limit, some say, of around 2000 characters. For more than that, we’ll use the also badly named POST method. POST has an upload limit on most servers of 8 megabytes. If you need more, server settings can be adjusted.
Let’s look at some code. You’ll see it looks very much like our last post:
Function btnAjax_onclick()
req=Ajax("ajaxPost.php","POST",txtSend.value)
If req.status=200 Then 'success
htmResponse.innerHTML=req.responseText
Else 'failure
htmResponse.innerHTML="Error: " & req.err
End If
End Function
The difference is a couple of extra parameters. We add “POST” and the data we want to send as a separate parameter. Before we talk about what is going to happen at the server end, let’s see what the app looks like:
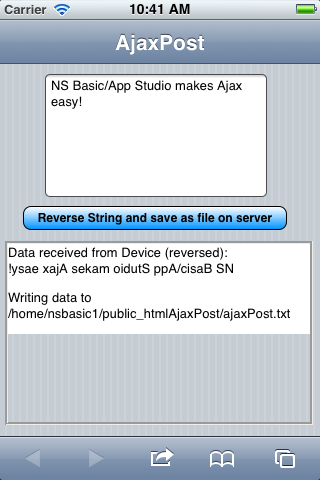
OK, here is the PHP code on the server which we are calling:
<?php
// uncomment the next line to see runtime error messages from this script
// ini_set('display_errors','1');
// Get the data from the client.
$myText = file_get_contents('php://input');
// Send back the text, reversed
echo "Data received from Device (reversed):<br>" . strrev($myText);
//write the data out to a file on the server
//make sure permissions are all OK!
$myFile = "AjaxPost/ajaxPost.txt";
echo "<p>Writing data to " . getcwd() . $myFile;
$f = fopen($myFile, 'w') or die("can't open file");
fwrite($f, $myText);
fclose($f);
?>
The file_get_contents(‘php://input’) call gets the data, exactly as it was sent. We’ll do two things with it: echo it back reversed and we will write it to a file on the server. The first part is easy: anything in an echo statement is sent back to our app:
echo "Data received from Device (reversed):
" . strrev($myText);
The second part involves a bit more PHP, but is straightforward. It writes the data was received to a file.
$myFile = "AjaxPost/ajaxPost.txt";
echo "<p>Writing data to " . getcwd() . $myFile;
$f = fopen($myFile, 'w') or die("can't open file");
fwrite($f, $myText);
fclose($f);
What would you use this feature for?
- Upload a JSON copy of your database.
- Upload some pictures in Base64 format.
- Create a .htm file which can be accessed as a web page.
- Lots more ideas!
A tip on debugging PHP scripts
Since PHP scripts run on the server and not on your screen, you don’t get to see the error messages. This can make debugging difficult. If you put this statement at the top of your PHP script, the error messages will be echoed to your app:
ini_set('display_errors','1');
The error messages come back as HTML formatted text. The best way to look at them is to assign them to the innerText property of an HTMLview control.